I’ve been speaking at various conferences recently about key skills for extending Office 365 – things that an effective architect or developer needs to have in the toolbox these days. Clearly the ability to work with the Microsoft Graph needs to be high on this list, given that it is after all, “the API for Office 365”. It’s great to have one (mainly!) consistent way to work with Teams, SharePoint, mail, calendar, Planner, OneDrive files and many other functions too, but between the various authentication options and coding styles, there’s quite a bit to know.
One thing I find is that developers are perhaps not effective enough with the Graph yet – partly because some things have changed recently, and partly because some devs are only now coming to the Graph from use of say, the SharePoint REST/CSOM APIs and other service-specific APIs.
So my key messages here are:
- The Postman collections provided by Microsoft can help answer your questions about the Graph and integrate calls into your code
- The SDKs help more than you’d expect in your code – having types in TypeScript being one example
Tip 1 - Using Postman collections instead of Graph Explorer
Something that makes Postman very powerful with the Graph is that Microsoft have released Postman collections for all the current Graph methods – including those in beta. You can import these into Postman and you’ll then see an item for every method, broken into two folders for app-only calls and on-behalf-of-user calls:
- Import the collections
- Configure various Postman variables
- Obtain access tokens for app-only and "on behalf of user" calls, and store in other Postman variables
- Enjoy your new quick access to the Graph! You can start executing the calls and seeing data coming back at this point
It's fairly straightforward to integrate the call into your code, and you won't have any security/permission issues because you've already tested the same thing your code will do.
UPDATE - actually, I realise now that Jeremy also has a video. See https://www.youtube.com/watch?v=4tg-OBdv_8o for that one.
So that's great - we can now see all the URL endpoints in the Graph, what data they expect and what data they return. But how do we ensure we're working effectively with the Graph once we're in code?
Tip 2 - ensure you're using TypeScript types
For many of us, the place that we'll be coding against the Graph will be SPFx or other TypeScript code - perhaps even an Azure Function based on node.js. The key thing to avoid here is use of “any” in TypeScript – we can do better than that, whether it’s another 3rd party API or the Graph itself. It’s common for APIs to return fairly complex JSON structures, and we can avoid lots of coding errors by ensuring we get type-checking through the use of types (interfaces or classes) representing the data. Additionally, having auto-complete against these data structures makes the coding process much faster and more accurate.
Using the Graph in TypeScript
When using the Graph in SPFx, the first thing to note is that your project will not have the Graph types added automatically (e.g. when the Yeoman generator creates the files) – you have to install a separate npm package. To do this, run one of the following:- npm install @microsoft/microsoft-graph-types --save-dev
- npm install @microsoft/microsoft-graph-types-beta --save-dev
It’s a good idea to only import specific entities that you’re actually using rather than *, but that needs some digging (to find the module name). You’ll be rewarded with a smaller bundle size however. Either way, once you’ve got the types imported you can start using them in your code – this changes things from having no zero support at all:
..to having full auto-complete as you type:
Hooray! Things are now much easier.
Wherever possible, don't settle for coding without this kind of support! But what if you're working with an API that isn't the Graph?
Using 3rd party APIs in TypeScript
The first thing to do in this case is establish if the vendor supplies TypeScript types (usually in the form of a npm package or similar). If so, just install that and you should get the support. If not, I like to use Postman to obtain the JSON returned and then use a Visual Studio Code extension such as QuickType to auto-generate types for me, based on that JSON. So you’d make the call to the API in Postman, and then copy the returned JSON to your clipboard.In the example below, I'm using the Here Maps API. I set up the call in Postman by pasting in the URL endpoint to use, and setting up any authentication. I hit the send button, and then copy the returned JSON (in the lower pane) to my clipboard):
In Visual Studio code, I create a file to hold the interface types from the API, and then find the QuickType "paste JSON as code" option in my command palette:
..and then QuickType generates the type structure which maps to the JSON. I might want to rename some of the types, but essentially I get interfaces for everything in the structure:
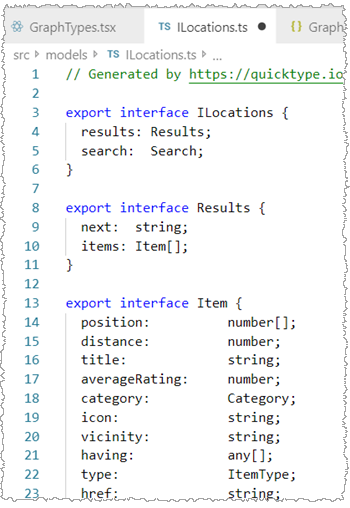
Summary
Whether it's the Graph or a 3rd party API, you should always code against defined TypeScript interfaces where possible. For the Graph, you should use Microsoft's supplied types by installing their 'microsoft-graph-types' npm package. For a 3rd party API, if no types are supplied then you can generate your own easily with help from something like QuickType.
Whatever you're doing, Postman is a hugely useful tool. In terms of the Graph, it's use gets you around the problem of Graph Explorer not executing with the same permissions as your code, and so is as valuable there as it is with 3rd party APIs.
Happy coding!
No comments:
Post a Comment